SESSION MODES IN ASP.NET:
ASP.NET provides various Session Modes for storage of Session Data. Here is the different session modes available in ASP.NET.
- InProc mode: This is the default Session State modes which store the session data in memory on the web server.
- StateServer (Out-Proc) mode: This stores the session data in separate memory called the ASP.NET Service. This mode ensures that the session data preserves when the Application Process restarts.
- SQL Server mode: In this mode session data is stored in the SQL Server Database. This mode also ensures that the session data preserves when the Web Application restarted. Also, this mode makes session data available to several Web Server.
- Custom mode: This mode enables you to specify the custom storage option.
- Off mode: This mode disables the session state. This increases the performance of the application.
Load Balance Example in Session:
InProcMode:
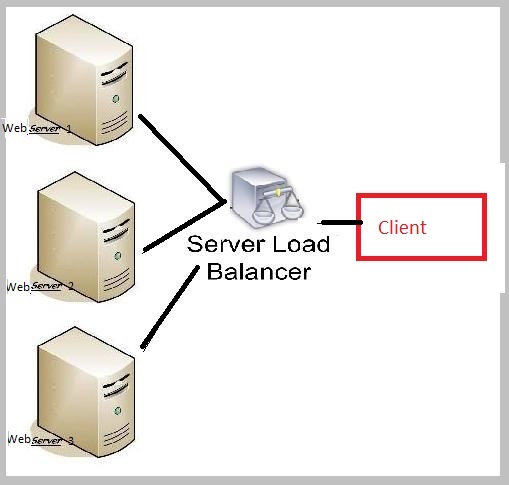
OutProc Mode:
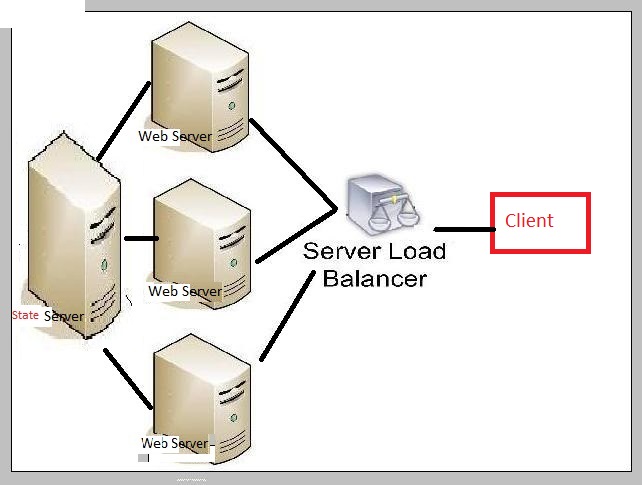
InProcMode:
OutProc Mode:
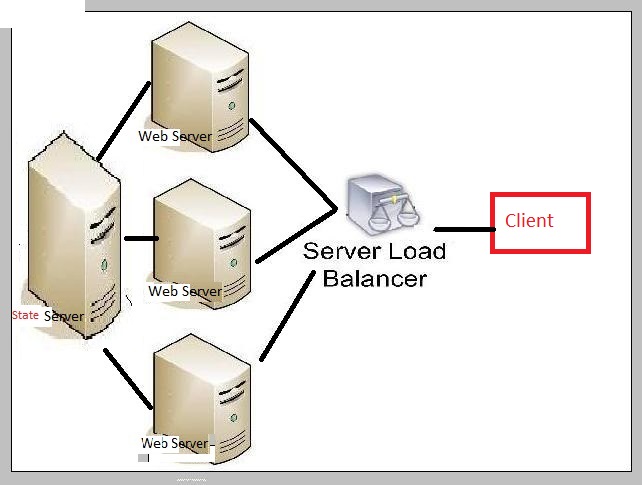
ViewState:
The ViewState property provides a dictionary object for retaining values between multiple requests for the same page. When an ASP.NET page is processed, the current state of the page and controls is hashed into a string and saved in the page as a hidden field. If the data is too long for a single field, then ASP.NET performs view state chunking (new in ASP.NET 2.0) to split it across multiple hidden fields. The following code sample demonstrates how view state adds data as a hidden form within a Web page’s HTML:
The ViewState property provides a dictionary object for retaining values between multiple requests for the same page. When an ASP.NET page is processed, the current state of the page and controls is hashed into a string and saved in the page as a hidden field. If the data is too long for a single field, then ASP.NET performs view state chunking (new in ASP.NET 2.0) to split it across multiple hidden fields. The following code sample demonstrates how view state adds data as a hidden form within a Web page’s HTML:
<input type="hidden" name="__VIEWSTATE" id="__VIEWSTATE” value="/wEPDwUKMTIxNDIyOTM0Mg9kFgICAw9kFgICAQ8PFgIeBFRleHQFEzQvNS8yMDA2IDE6Mzc6MTEgUE1kZGROWHn/rt75XF/pMGnqjqHlH66cdw==" />
Encrypting of the View State: You can enable view state encryption to make it more difficult for attackers and malicious users to directly read view state information. Though this adds processing overhead to the Web server, it supports in storing confidential information in view state. To configure view state encryption for an application does the following:
Cookies:
Controlling the Cookie Scope: By default, browsers won’t send a cookie to a Web site with a different hostname. You can control a cookie’s scope to either limit the scope to a specific folder on the Web server or expand the scope to any server in a domain. To limit the scope of a cookie to a folder, set the Path property, as the following example demonstrates:
Example:
Response.Cookies["lastVisit"].Path = "/Application1";
Through this the scope is limited to the “/Application1” folder that is the browser submits the cookie to any page with in this folder and not to pages in other folders even if the folder is in the same server. We can expand the scope to a particular domain using the following statement:
Example:
Response.Cookies[“lastVisit”].Domain = “Contoso”;
Storing Multiple Values in a Cookie:
Though it depends on the browser, you typically can’t store more than 20 cookies per site, and each cookie can be a maximum of 4 KB in length. To work around the 20-cookie limit, you can store multiple values in a cookie, as the following code demonstrates:
Example:
Response.Cookies["info"]["visit"].Value = DateTime.Now.ToString(); Response.Cookies["info"]["firstName"].Value = "Tony"; Response.Cookies["info"]["border"].Value = "blue"; Response.Cookies["info"].Expires = DateTime.Now.AddDays(1);
https://www.c-sharpcorner.com/UploadFile/de41d6/view-state-vs-session-state-vs-application-state/