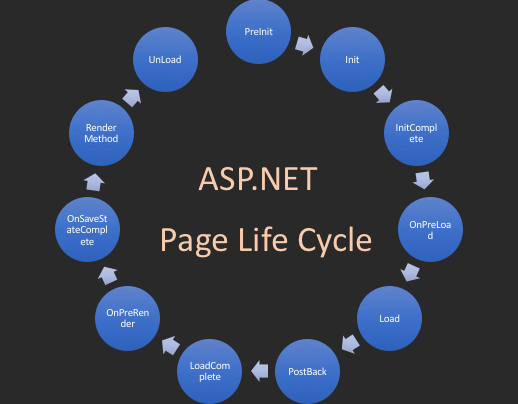
Preinit:
Check the IsPostBack property to determine whether this is the first time the page is being processed.
Create or re-create dynamic controls.
Set a master page dynamically.
Set the Theme property dynamically.
Note:
If the request is a postback then the values of the controls have not yet been restored from the view state.
If you set a control property at this stage, its value might be overwritten in the next event.
Init:
- This event fires after each control has been initialized.
- Each control's UniqueID is set and any skin settings have been applied.
- Use this event to read or initialize control properties.
- The "Init" event is fired first for the bottom-most control in the hierarchy, and then fired up the hierarchy until it is fired for the page itself.
InitComplete:
Until now the viewstate values are not yet loaded, hence you can use this event to make changes to the view state that you want to ensure are persisted after the next postback.
Raised by the Page object.
Use this event for processing tasks that require all initialization to be complete.
OnPreLoad:
Loads ViewState: ViewState data are loaded to controls.
Loads Postback data: Postback data are now handed to the page controls.
Load:
This is the first place in the page lifecycle that all values are restored.
- Most code checks the value of IsPostBack to avoid unnecessarily resetting state.
- You may also call Validate and check the value of IsValid in this method.
- You can also create dynamic controls in this method.
Control PostBack Event(s):
- Use these events to handle specific control events, such as a Button control's Click event or a TextBox control's TextChanged event.
- In a postback request, if the page contains validator controls, check the IsValid property of the Page and of individual validation controls before performing any processing.
- This is just an example of a control event. Here it is the button click event that caused the postback.
LoadComplete:
- Raised at the end of the event-handling stage.
- Use this event for tasks that require that all other controls on the page be loaded.
OnPreRender:
- Allows final changes to the page or its control.
- This event takes place before saving ViewState, so any changes made here are saved.
- For example: After this event, you cannot change any property of a button or change any viewstate value.
- Each data bound control whose DataSourceID property is set calls its DataBind method.
- Use the event to make final changes to the contents of the page or its controls.
OnSaveStateComplete:
- Raised after view state and control state have been saved for the page and for all controls.
- Before this event occurs, ViewState has been saved for the page and for all controls.
- Any changes to the page or controls at this point will be ignored.
- Use this event perform tasks that require the view state to be saved, but that do not make any changes to controls.
Render Method:
- The Render method generates the client-side HTML, Dynamic Hypertext Markup Language (DHTML), and script that are necessary to properly display a control at the browser.
UnLoad:
- This event is used for cleanup code.
- At this point, all processing has occurred and it is safe to dispose of any remaining objects, including the Page object.